Vue Js Conditionally Enable Disable Button:In Vue.js, you can conditionally enable or disable a button by using the :disabled
directive along with a boolean variable. Firstly, define a data property, let’s say isButtonEnabled
, and set it to true
or false
based on your condition. Then, in your button element, use the :disabled
directive and bind it to the isButtonEnabled
variable. For example, <button :disabled="!isButtonEnabled">Submit</button>
. This will enable the button when isButtonEnabled
is true
and disable it when it’s false
. You can dynamically update isButtonEnabled
in response to user actions or other conditions in your Vue component.
How can I Vue Js conditionally enable disable button?
In the provided Vue.js code, a select
element with three options and a button
element are defined inside a div
with the id “app”. The button
element has a :disabled
attribute that binds to a computed property called isButtonDisabled
.
The data
section of the Vue app contains a property called selectedValue
, which represents the currently selected option in the select
element. Initially, no value is selected.
The computed
section defines a computed property named isButtonDisabled
. This property returns true
if no value is selected (this.selectedValue === ''
), which means the button will be disabled. If a value is selected, the property returns false
, enabling the button.
By using this setup, the button’s disabled state is updated dynamically based on the selected option in the select
element.
Vue Js Conditionally Enable Disable Button Example
<div id="app">
<select v-model="selectedValue">
<option value="option1">Option 1</option>
<option value="option2">Option 2</option>
<option value="option3">Option 3</option>
</select>
<button :disabled="isButtonDisabled">Submit</button>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
selectedValue: '', // Initially no value is selected
};
},
computed: {
isButtonDisabled() {
return this.selectedValue === ''; // Disable the button if no value is selected
},
},
});
app.mount('#app');
</script>
Output of Vue Js Conditionally Enable Disable Button
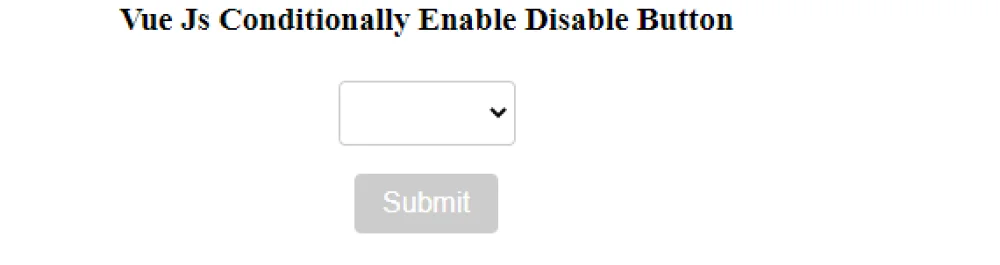